int r = base+random.nextInt(max);
return String.fromCharCode(r);
}
* 异步遍历
await Future.forEach(list, (element) => null);
Stream<FileSystemEntity> fileList = directory.list(followLinks: false); // 列出文件夹内文件,返回值为异步对象
await fileList.forEach((e) => null);
flutter相关
=========
有话要说
----
受前端思维影响,奉劝各位入门的前端人员: **抛弃前端的思维去学习flutter**
1. 在flutter中,整体长度和宽度是定死的,就是屏幕的长度和宽度,(width=MediaQuery.of(context).size.width,同理获取高度,高度包含状态栏) 。
2. 不存在溢出自动滚动,所以你在堆组件的时候要注意,最大高度等于屏幕高度,超出就报错,或者用滚动组件**SingleChildScrollView**等作为根元素。
3. 不存在百分比长度宽度,容器的width和height可以不设置,设置就要有明确的数值,什么?就是想要百分比,那么有2个方法:
(1)屏幕宽高度/*百分比 ,(2) 使用**row**或者**column**组件,里面**Expanded**组件有个参数**flex**,跟前端类似,实现比例分配。
判断当前是debug还是release
-------------------
static const bool isProduction = const bool.fromEnvironment("dart.vm.product");
热加载失效
-----
如果你在idea中使用鼠标拖拽移动了文件路径,那么恭喜你这个文件的热加载失效了。
原因是文件路径变成了绝对路径,类似C://Users...,这种路径热加载识别不了,需改为package:...
点击空白无效
------
(1)**GestureDetector**设置参数 **behavior** 为 **HitTestBehavior.opaque**,
(2)使用**InkWell**组件
键盘溢出
----
默认情况下,键盘弹起,flutter会将页面上推,可能会导致溢出报错,解决办法有两个:
1. 修改默认值
Scaffold(
appBar: AppBar(
title: new Text("首页"),
),
resizeToAvoidBottomPadding: false, //默认值为true,表示页面上推, 设置false表示不上推,此时键盘可能会盖住页面,类似stack层叠效果
);
2. 使用滚动组件
使用**SingleChildScrollView**或者**listview**组件作为根元素,此时就不要设置**resizeToAvoidBottomPadding**为false了,要不然就没有页面上推了
showModalBottomSheet 底部弹出 问题
----------------------------
* 顶部圆角
shape: RoundedRectangleBorder(borderRadius: BorderRadius.vertical(top: Radius.circular(20))),
* 高度限制
默认高度为半屏,设置**isScrollControlled**为true是全屏,不想全屏,使用**BoxConstraints**组件限制最大高度
* 键盘溢出
首先使用**SingleChildScrollView**作为根组件让其可以滚动,然后获取键盘高度**MediaQuery.of(context).viewInsets.bottom**作为**padding**的**bottom**,因为这不在**scaffold**工作范围内,flutter不会为我们上推界面。  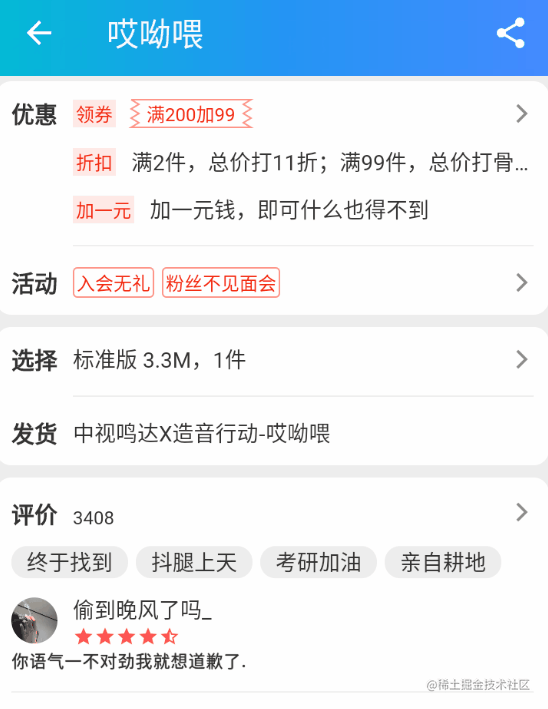
* 状态更新
**showModalBottomSheet**等其他dialog组件,相当于跳转的一个新的路由页面,在这个页面**setState(() {});** 更新上个页面的状态没有用。
解决办法有许多,其中一个是使用**Builder**组件包裹要更新的组件,在更新时调用 **(context as Element).markNeedsBuild();** 
TextField 内容垂直不居中
-----------------
contentPadding: EdgeInsets.all(0.0),
去除水波纹
-----
默认情况下,可滚动组件滑到顶部和尾部会有水波纹效果,如图所示,那么怎么去掉呢?
全局去掉如下:
MaterialApp(
builder: (context, child) {
child= ScrollConfiguration(
child: child,
behavior: RefreshScrollBehavior(),
);
return child;
},
)
class RefreshScrollBehavior extends ScrollBehavior {
@override
Widget buildViewportChrome(
BuildContext context, Widget child, AxisDirection axisDirection) {
switch (getPlatform(context)) {
case TargetPlatform.iOS:
return child;
case TargetPlatform.macOS:
case TargetPlatform.android:
return GlowingOverscrollIndicator(
child: child,
showLeading: false, //顶部水波纹是否展示
showTrailing: false, //底部水波纹是否展示
axisDirection: axisDirection,
notificationPredicate: (notification) {
if (notification.depth == 0) {
// 越界是否展示水波纹
if (notification.metrics.outOfRange) {
return false;
}
return true;
}
return false;
},
color: Theme.of(context).primaryColor,
);
case TargetPlatform.fuchsia:
}
return null;
}
}
渐变appbar
通过设置AppBar的flexibleSpace属性
flexibleSpace: Container(
decoration: BoxDecoration(
gradient: LinearGradient(
colors: [Colors.cyan, Colors.blue, Colors.blueAccent],
),
),
),
动态渐变appbar
使用NotificationListener监听页面滚动,动态改变appbar透明值。
body: NotificationListener(
onNotification: (scrollNotification) {
if (scrollNotification is ScrollUpdateNotification) {
if (scrollNotification.metrics.axis == Axis.vertical) _onScroll(scrollNotification.metrics.pixels);
}
return false;
},
_onScroll(offset) {
//print(offset);
if (offset > 200) return;
double alpha = offset / 200;
if (alpha < 0) {
alpha = 0;
} else if (alpha > 1) {
alpha = 1;
}
setState(() {
appBarAlpha = alpha;
});
}
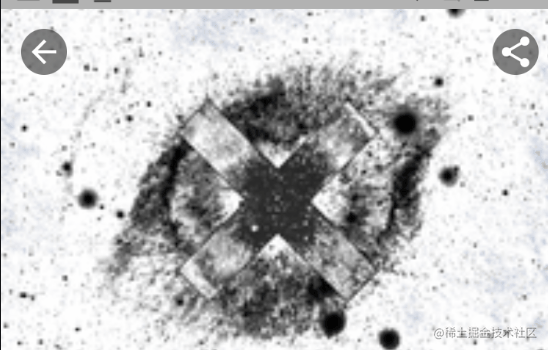
自适应宽高
-----
使用**FittedBox**组件可自动调节内容,超出宽高会自动调节字体大小
自定义底部导航
-------
如图所示,这种导航条官方没有提供,只能靠我们自定义了。 通过自定义**Scaffold**的**bottomNavigationBar**属性来实现,其中bottomAppBarItem是一个自定义方法,生成一个个导航按钮,红点使用**stack**相对定位,中间是一个播放进度按钮,类似喜马拉雅,思路是**CircularProgressIndicator**组件作为进度条,**Container**组件形状指定为圆 **shape: BoxShape.circle**,子组件是图片,然后相对定位于**CircularProgressIndicator**
bottomNavigationBar: BottomAppBar(
child: Consumer<IMNoticeProvider>(
builder: (context,_imNotice,child){
return Row(
children: [
bottomAppBarItem(0, Icons.home, ‘首页’, badge: badge1),
bottomAppBarItem(1, Icons.email, ‘消息’, badge: _imNotice.unreadMsgCount),
bottomAppBarItem(-1, Icons.store, ‘商店’, badge: badge1),
bottomAppBarItem(2, Icons.store, ‘商店’, badge: 101),
bottomAppBarItem(3, Icons.person, ‘我的’, badge: 1, type: ‘q’),
],
mainAxisAlignment: MainAxisAlignment.spaceAround, //均分底部导航栏横向空间
mainAxisSize: MainAxisSize.max,
);
},
)
)
 
popupMenu 弹出菜单 滑动关闭
-------------------
官方的弹出菜单,需要点击空白才能关闭,如何才能滑动屏幕就能关闭呢?参照微信长按聊天会话。
官方没有提供,只能我们自定义了。
复制showMenu函数源码到项目文件夹下,并更名为customShowMenu,防止与官方冲突,用法不变。
大约在770行,添加**GestureDetector**组件,我们自己处理滑动事件。
return MediaQuery.removePadding(
context: context,
removeTop: true,
removeBottom: true,
removeLeft: true,
removeRight: true,
child: GestureDetector(
behavior: HitTestBehavior.translucent,
onPanStart: (DragStartDetails details) {
Navigator.of(context).maybePop();
},
child: Builder(
builder: (BuildContext context) {
return CustomSingleChildLayout(
delegate: _PopupMenuRouteLayout(
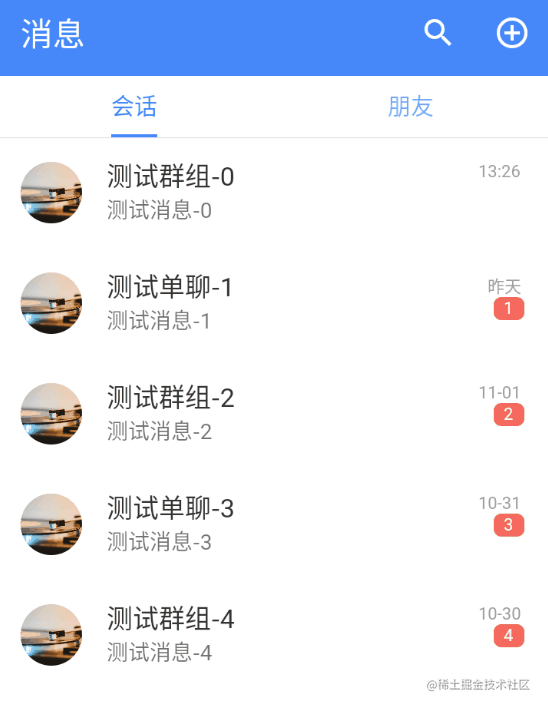
tabbar 保存位置
-----------
默认情况下,tabbar切换,上一个页面滚动的位置会销毁, 解决办法:使用key保存位置
var _tab1 = PageStorageKey(‘_tab1’);
自定义搜索
-----
如图所示 ,官方自带搜索组件**showSearch**,需要实现一个**SearchDelegate**,为了实现底部tabbar,我们需要修改源码。
复制**SearchDelegate** 源码到我们项目文件夹下,并更名为myShowSearchGoods和MySearchDelegateGoods,名字随意防止与官方冲突,这个一个抽象类,后面我们实现它。
GestureDetector(
behavior: HitTestBehavior.opaque,
onTap: () => myShowSearchGoods(context: context, delegate: GoodsSearchBarDelegate()),
child: Container(
class GoodsSearchBarDelegate extends MySearchDelegateGoods<String> {
List recentSuggest = List.from(MySheetSearch.getData().reversed.toList());
int id = 0;
//List tabTitle = ['单曲', '专辑', '歌手', '歌单'];
List songList = [];
List albumList = [];
List artistList = [];
List sheetList = [];
int page1,page2,page3,page4=0;
List tabTitle = [
{"name": "单曲", "type": 1},
{"name": "专辑", "type": 10},
{"name": "歌手", "type": 100},
{"name": "歌单", "type": 1000},
];
String oldQuery;
RefreshController _controllerR1 =RefreshController(initialRefresh: false);
RefreshController _controllerR2 =RefreshController(initialRefresh: false);
RefreshController _controllerR3 =RefreshController(initialRefresh: false);
RefreshController _controllerR4 =RefreshController(initialRefresh: false);
GoodsSearchBarDelegate();
@override
String get searchFieldLabel => '搜点什么';
@override
loadData(BuildContext context) async { //加载数据
if(query.isEmpty){
Utils.showToast('请输入搜索内容');
return false;
}
if (oldQuery != query) {
oldQuery = query;
songList = [];
albumList = [];
artistList = [];
sheetList = [];
page1=0;
page2=0;
page3=0;
page4=0;
}
else
showResults(context);
if (tabController.index == 0 && (songList==null || songList.isNotEmpty))
return false;
else if (tabController.index == 1 && (albumList==null || albumList.isNotEmpty)) return false;
else if (tabController.index == 2 && (artistList==null || artistList.isNotEmpty)) return false;
else if (tabController.index == 3 && (sheetList==null || sheetList.isNotEmpty)) return false;
var cancel = Utils.showLoading();
List data = await GoodsSearch().getSearchRes(query, type: tabTitle[tabController.index]['type']);
cancel();
if (tabController.index == 0) songList = data;
else if (tabController.index == 1) albumList = data;
else if (tabController.index == 2) artistList = data;
else if (tabController.index == 3) sheetList = data;
showResults(context);
}
loadMoreData(int page) async{
// var cancel = Utils.showLoading();
List data = await GoodsSearch().getSearchRes(query, type: tabTitle[tabController.index]['type'],page: page);
// cancel();
return data;
}
@override
Widget buildAppBarBottom(BuildContext context) { //tabbar
return PreferredSize(
preferredSize: Size.fromHeight(40.0),
### 最后
这里附上上述的技术体系图相关的几十套**腾讯、头条、阿里、美团等公司2021年的面试题**,把技术点整理成了视频和PDF(实际上比预期多花了不少精力),包含**知识脉络 + 诸多细节**,由于篇幅有限,这里以图片的形式给大家展示一部分。
**相信它会给大家带来很多收获:**

上述**高清技术脑图**以及**配套的架构技术PDF**可以[点击我的腾讯文档免费获取](https://gitee.com/vip204888/android-p7)
当程序员容易,当一个优秀的程序员是需要不断学习的,从初级程序员到高级程序员,从初级架构师到资深架构师,或者走向管理,从技术经理到技术总监,每个阶段都需要掌握不同的能力。早早确定自己的职业方向,才能在工作和能力提升中甩开同龄人。
- 无论你现在水平怎么样一定要 持续学习 没有鸡汤,别人看起来的毫不费力,其实费了很大力,这四个字就是我的建议!!!
- 我希望每一个努力生活的IT工程师,都会得到自己想要的,因为我们很辛苦,我们应得的。
> 当我们在抱怨环境,抱怨怀才不遇的时候,没有别的原因,一定是你做的还不够好!
原创文章,作者:ItWorker,如若转载,请注明出处:https://blog.ytso.com/115651.html